Inheritance is the process of creating new classes from the existing class or classes.
Using inheritance, one can create general class that defines traits common to a set of related items. This class can then be inherited (reused) by the other classes by using the properties of the existing ones with the addition of its own unique properties.
The old class is referred to as the base class and the new classes, which are inherited from the base class, are called derived classes.
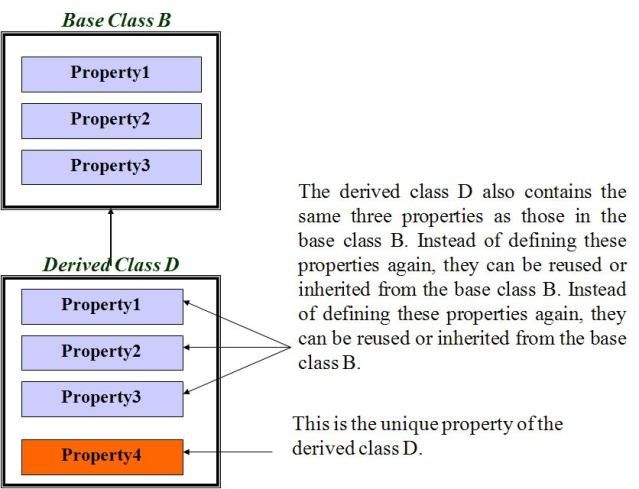
In above figure, the class B contains the three member i.e. Property1, Property2 and Property3.
The second class named as D contains four members i.e. Property1, Property2, Property3 and Property4.
Out of these, three members (Property1, Property2, Property3) of the class D are same as that of class B, while the one member i.e. Property4 is unique.
In the class D, instead of defining all the three members again, one can reuse them from the class B. This feature is known as inheritance which saves time, space, money and increases the reliability and efficiency.
Forms of Inheritance:
Single Inheritance
If a class is derived from a single base class, it is called as single inheritance.
Multiple Inheritance
If a class is derived from more than one base class, it is known as multiple inheritance
Multilevel Inheritance
The classes can also be derived from the classes that are already derived. This type of inheritance is called multilevel inheritance.
Hierarchical Inheritance
If a number of classes are derived from a single base class, it is called as hierarchical inheritance
A derived class can be defined as follows:
class derived_class_name : access_specifier base_class_name
{
data members of the derived class ;
member functions of the derived class ;
}
The colon (:), indicates that the class derived_class_name is derived from the class base_class_name.
The access_specifier may be public, private or protected (will be discussed further).
If no access_specifier is specified, it is private by default.
The access_specifier indicates whether the members of the base class are privately derived or publicly derived.
Public inheritance
When a derived class publicly inherits the base class, all the public members of the base class also become public to the derived class and the objects of the derived class can access the public members of the base class.
The following program will illustrate the use of the single inheritance.
This program has a base class B, from which class D is inherited publicly.
Example:
#include
class Rectangle
{
private:
float length ; // This can't be inherited
public:
float breadth ; // The data and member functions are inheritable
void Enter_lb(void)
{
cout << "\n Enter the length of the rectangle : ";
cin >> length ;
cout << "\n Enter the breadth of the rectangle : ";
cin >> breadth ;
}
float Enter_l(void)
{ return length ; }
}; // End of the class definition
class Rectangle1 : public Rectangle
{
private:
float area ;
public:
void Rec_area(void)
{ area = Enter_l( ) * breadth ; }
// area = length * breadth ; can't be used here
void Display(void)
{
cout << "\n Length = " << Enter_l( ) ; // Object of the derived class can't
// inherit the private member of the base class. Thus the member
// function is used here to get the value of data member 'length'.
cout << "\n Breadth = " << breadth ;
cout << "\n Area = " << area ;
}
}; // End of the derived class definition D
void main(void)
{
Rectangle1 r1 ;
r1.Enter_lb( );
r1.Rec_area( );
r1.Display( );
}
Figure- Public Inheritance
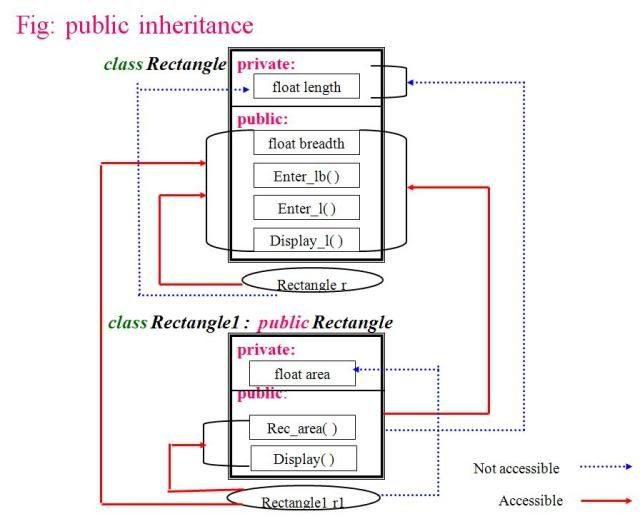
Private inheritance
When a derived class privately inherits a base class, all the public members of the base class become private for the derived class.
In this case, the public members of the base class can only be accessed by the member functions of the derived class.
The objects of the derived class cannot access the public members of the base class.
Note that whether the derived class is inherited publicly or privately from the base class, the private members of the base class cannot be inherited.
Example:
#include
#include
class Rectangle
{
int length, breadth;
public:
void enter()
{
cout << "\n Enter length: "; cin >> length;
cout << "\n Enter breadth: "; cin >> breadth;
}
int getLength()
{
return length;
}
int getBreadth()
{
return breadth;
}
void display()
{
cout << "\n Length= " << length;
cout << "\n Breadth= " << breadth;
}
};
class RecArea : private Rectangle
{
public:
void area_rec()
{
enter();
cout << "\n Area = " << (getLength() * getBreadth());
}
};
void main()
{
clrscr();
RecArea r ;
r.area_rec();
getch();
}
Example:2
#include
class Rectangle // Base class
{
private:
float length ; // This data member can't be inherited
public:
float breadth ; // This data member is inheritable
void Enter_lb(void)
{
cout << "\n Enter the length of the rectangle: "; cin >> length ;
cout << "\n Enter the breadth of the rectangle: "; cin >> bredth ;
}
float Enter_l(void)
{
return length ;
}
void Display_l(void)
{
cout << "\n Length = " << length ;
}
}; // End of the base class Rectangle.
// Defining the derived class Rectangle1. This class has been derived from the
// base class i.e. Rectangle, privately.
class Rectangle1 : private Rectangle // All the public members of the base class
{ // Rectangle become private for the derived class Rectangle1.
private:
float area ;
public:
void Rec_area(void)
{
Enter_lb( );
area = Enter_l( ) * bredth ; // length can't be used directly
}
void Display(void)
{
Display_l( ); // Displays the value of length.
cout << "\n Bredth = " << bredth ;
cout << "\n Area = " << area << endl ;
}
};
void main(void)
{
Rectangle1 r1 ;
r1.Rec_area( ) ;
// r.Enter_lb( ); will not work as it has become private for the derived class.
r1.Display( ) ;
// r.Display_l( ) will not work as it also has become private for the derived class.
}
Fig: private inheritance
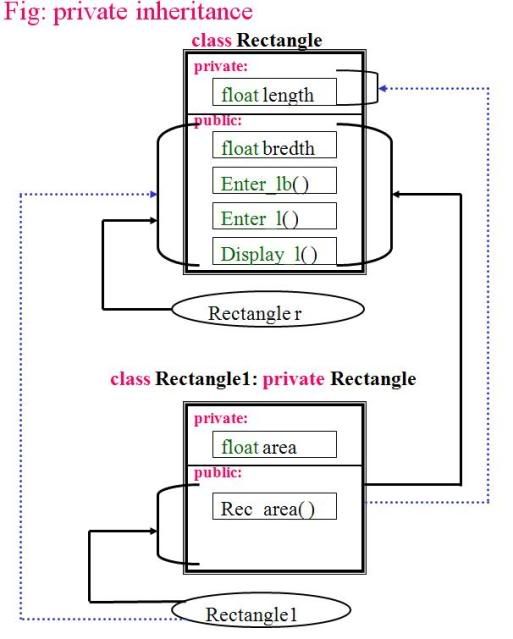
The protected access specifier
The third access specifier provided by C++ is protected.
The members declared as protected can be accessed by the member functions within their own class and any other class immediately derived from it.
These members cannot be accessed by the functions outside these two classes.
Therefore, the objects of the derived class cannot access protected members of the base class.
When the protected members (data, functions) are inherited in public mode, they become protected in the derived class. Thus, they can be accessed by the member functions of the derived class.
On other hand, if the protected members are inherited in the private mode, the members also become private in the derived class.
They can also be accessed by the member functions of the derived class, but cannot be inherited further.
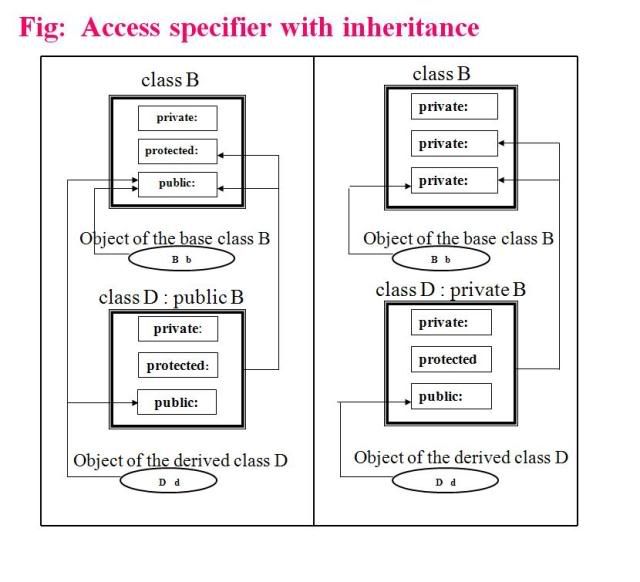
Overriding the member functions:
The member functions can also be used in a derived class, with the same name as those in the base class.
One might want to do this so that calls in the program work the same way for objects of both base and derived classes.
The following program will illustrate this concept.
#include
const int len = 20 ;
class Employee
{
private:
char F_name[len];
int I_number ;
int age ;
float salary ;
public:
void Enter_data(void)
{
cout << "\n Enter the first name = " ; cin >> F_name ;
cout << "\n Enter the identity number = " ; cin >> I_number ;
cout << "\n Enter the age = " ; cin >> age ;
cout << "\n Enter the salary = " ; cin >> salary ;
}
void Display_data(void)
{
cout << "\n Name = " << F_name ;
cout << "\n Identity Number = " << I_number ;
cout << "\n Age = " << age ;
cout << "\n Salary = " << salary ;
}
}; // End of the base class
class Engineer : public Employee
{
private:
char design[len] ; // S_Engineer, J_Engineer, Ex_Engineer etc
public:
void Enter_data( )
{
Employee :: Enter_data( ) ; // Overriding of the member function
cout << "\n Enter the designation of the Engineer: " ; cin >> design ;
}
void Display_data(void)
{
cout << "\n *******Displaying the particulars of the Engineer**** \n" ;
Employee :: Display_data( ) ; // Overriding of the member function
cout << "\n Designition = " << design ;
}
}; // End of the derived class
void main(void)
{
Engineer er ;
er.Enter_data( ) ;
er.Display_data( );
}
Hierarchical Inheritance:
When two or more classes are derived from a single base class, then
Inheritance is called the hierarchical inheritance. The representation
of the hierarchical inheritance is shown in the following Fig.
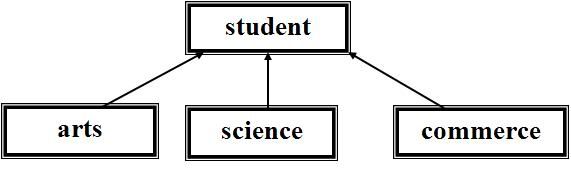
In the above Fig., student is a base class, from which the three classes viz. arts, science and commerce have been derived. Now, let us write a program that illustrates the hierarchical inheritance, based on the above design.
Example
include
const int len = 20 ;
class student // Base class
{
private: char F_name[len] , L_name[len] ;
int age, int roll_no ;
public:
void Enter_data(void)
{
cout << "\n\t Enter the first name: " ; cin >> F_name ;
cout << "\t Enter the second name: "; cin >> L_name ;
cout << "\t Enter the age: " ; cin >> age ;
cout << "\t Enter the roll_no: " ; cin >> roll_no ;
}
void Display_data(void)
{
cout << "\n\t First Name = " << F_name ;
cout << "\n\t Last Name = " << L_name ;
cout << "\n\t Age = " << age ;
cout << "\n\t Roll Number = " << roll_no ;
}
};
class arts : public student
{
private:
char asub1[len] ;
char asub2[len] ;
char asub3[len] ;
public:
void Enter_data(void)
{
student :: Enter_data( );
cout << "\t Enter the subject1 of the arts student: "; cin >> asub1 ;
cout << "\t Enter the subject2 of the arts student: "; cin >> asub2 ;
cout << "\t Enter the subject3 of the arts student: "; cin >> asub3 ;
}
void Display_data(void)
{
student :: Display_data( );
cout << "\n\t Subject1 of the arts student = " << asub1 ;
cout << "\n\t Subject2 of the arts student = " << asub2 ;
cout << "\n\t Subject3 of the arts student = " << asub3 ;
}
};
class commerce : public student
{
private: char csub1[len], csub2[len], csub3[len] ;
public:
void Enter_data(void)
{
student :: Enter_data( );
cout << "\t Enter the subject1 of the commerce student: ";
cin >> csub1;
cout << "\t Enter the subject2 of the commerce student: ";
cin >> csub2 ;
cout << "\t Enter the subject3 of the commerce student: ";
cin >> csub3 ;
}
void Display_data(void)
{
student :: Display_data( );
cout << "\n\t Subject1 of the commerce student = " << csub1 ;
cout << "\n\t Subject2 of the commerce student = " << csub2 ;
cout << "\n\t Subject3 of the commerce student = " << csub3 ;
}
};
void main(void)
{
arts a ;
cout << "\n Entering details of the arts student\n" ;
a.Enter_data( );
cout << "\n Displaying the details of the arts student\n" ;
a.Display_data( );
science s ;
cout << "\n\n Entering details of the science student\n" ;
s.Enter_data( );
cout << "\n Displaying the details of the science student\n" ;
s.Display_data( );
commerce c ;
cout << "\n\n Entering details of the commerce student\n" ;
c.Enter_data( );
cout << "\n Displaying the details of the commerce student\n";
c.Display_data( );
}
Multiple Inheritance
When a class is inherited from more than one base class, it is known as multiple inheritance.
The syntax for defining a subclass, which is inheriting more than one classes is:
class Subclass : access_specifier Baseclass1,
access_specifier Baseclass2, ………
…….. access_specifier Baseclass_n
{
members of the derived class ;
};
The following figure illustrates the use of multiple inheritance.
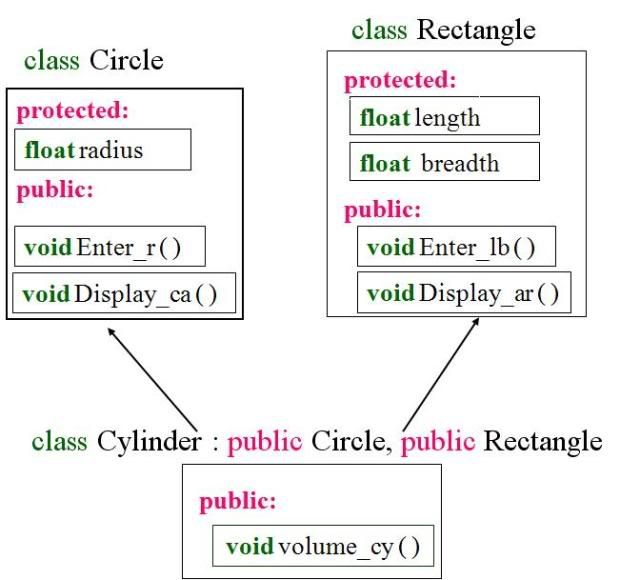
In the above figure, Circle and Rectangle are two base classes from which the class Cylinder is being inherited.
The data members of both the base classes are declared in protected mode. Thus, the class Cylinder can access the data member radius of class Circle and data member length, breadth of the class Rectangle, but the objects of the class Cylinder cannot access these protected data members.
The volume of the cylinder is equal to 22/7*(radius*radius*length). Thus, instead of defining these data again, they can be inherited from the base classes Circle and Rectangle ( radius from class Circle and length from class Rectangle ).
Example:
#include
class Circle // First base class
{
protected:
float radius ;
public:
void Enter_r(void)
{
cout << "\n\t Enter the radius: "; cin >> radius ;
}
void Display_ca(void)
{
cout << "\t The area = " << (22/7 * radius*radius) ;
}
};
class Rectangle // Second base class
{
protected:
float length, breadth ;
public:
void Enter_lb(void)
{
cout << "\t Enter the length : "; cin >> length ;
cout << “\t Enter the breadth : ” ; cin >> breadth ;
}
void Display_ar(void)
{
cout << "\t The area = " << (length * breadth);
}
};
class Cylinder : public Circle, public Rectangle // Derived class, inherited
{ // from classes Circle & Rectangle
public:
void volume_cy(void)
{
cout << "\t The volume of the cylinder is: "
<< (22/7* radius*radius*length) ;
}
};
void main(void)
{
Circle c ;
cout << "\n Getting the radius of the circle\n" ;
c.Enter_r( );
c.Display_ca( );
Rectangle r ;
cout << "\n\n Getting the length and breadth of the rectangle\n\n";
r.Enter_l( );
r.Enter_b( );
r.Display_ar( );
Cylinder cy ; // Object cy of the class cylinder which can access all the
// public members of the class circle as well as of the class rectangle
cout << "\n\n Getting the height and radius of the cylinder\n";
cy.Enter_r( );
cy.Enter_lb( );
cy.volume_cy( );
}
Multilevel Inheritance:
It has been discussed so far that a class can be derived from a class.
C++ also provides the facility of multilevel inheritance, according to which the derived class can also be derived by an another class, which in turn can further be inherited by another and so on.
The following figure will illustrate the meaning of the multilevel inheritance.
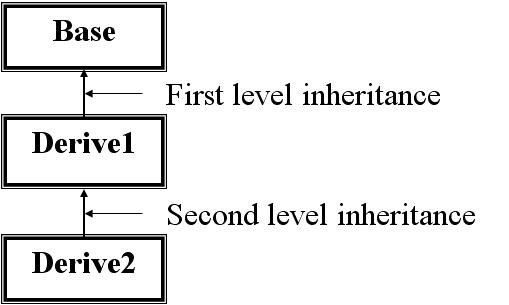
In the above figure, class B represents the base class. The class D1 that is called first level of inheritance, inherits the class B. The derived class D1 is further inherited by the class D2, which is called second level of inheritance.
Example: Multilevel Inheritance
#include
class Base
{
protected:
int b;
public:
void EnterData( )
{
cout << "\n Enter the value of b: ";
cin >> b;
}
void DisplayData( )
{
cout << "\n b = " << b;
}
};
class Derive1 : public Base
{
protected:
int d1;
public:
void EnterData( )
{
Base:: EnterData( );
cout << "\n Enter the value of d1: ";
cin >> d1;
}
void DisplayData( )
{
Base::DisplayData();
cout << "\n d1 = " << d1;
}
};
class Derive2 : public Derive1
{
private:
int d2;
public:
void EnterData( )
{
Derive1::EnterData( );
cout << "\n Enter the value of d2: ";
cin >> d2;
}
void DisplayData( )
{
Derive1::DisplayData( );
cout << "\n d2 = " << d2;
}
};
int main( )
{
Derive2 objd2;
objd2.EnterData( );
objd2.DisplayData( );
return 0;
}
Using inheritance, one can create general class that defines traits common to a set of related items. This class can then be inherited (reused) by the other classes by using the properties of the existing ones with the addition of its own unique properties.
The old class is referred to as the base class and the new classes, which are inherited from the base class, are called derived classes.
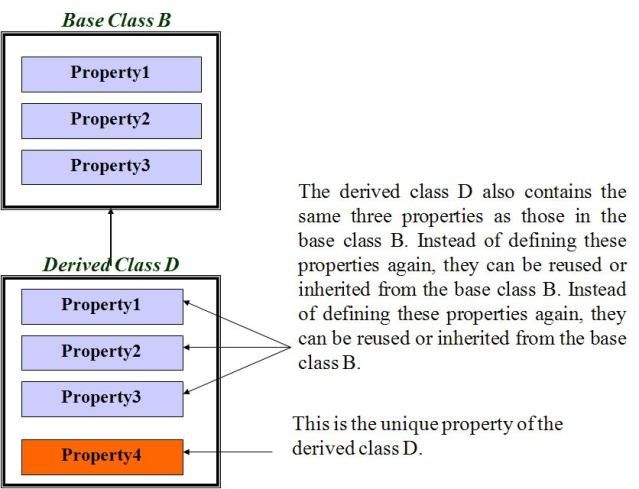
In above figure, the class B contains the three member i.e. Property1, Property2 and Property3.
The second class named as D contains four members i.e. Property1, Property2, Property3 and Property4.
Out of these, three members (Property1, Property2, Property3) of the class D are same as that of class B, while the one member i.e. Property4 is unique.
In the class D, instead of defining all the three members again, one can reuse them from the class B. This feature is known as inheritance which saves time, space, money and increases the reliability and efficiency.
Forms of Inheritance:
Single Inheritance
If a class is derived from a single base class, it is called as single inheritance.
Multiple Inheritance
If a class is derived from more than one base class, it is known as multiple inheritance
Multilevel Inheritance
The classes can also be derived from the classes that are already derived. This type of inheritance is called multilevel inheritance.
Hierarchical Inheritance
If a number of classes are derived from a single base class, it is called as hierarchical inheritance
A derived class can be defined as follows:
class derived_class_name : access_specifier base_class_name
{
data members of the derived class ;
member functions of the derived class ;
}
The colon (:), indicates that the class derived_class_name is derived from the class base_class_name.
The access_specifier may be public, private or protected (will be discussed further).
If no access_specifier is specified, it is private by default.
The access_specifier indicates whether the members of the base class are privately derived or publicly derived.
Public inheritance
When a derived class publicly inherits the base class, all the public members of the base class also become public to the derived class and the objects of the derived class can access the public members of the base class.
The following program will illustrate the use of the single inheritance.
This program has a base class B, from which class D is inherited publicly.
Example:
#include
class Rectangle
{
private:
float length ; // This can't be inherited
public:
float breadth ; // The data and member functions are inheritable
void Enter_lb(void)
{
cout << "\n Enter the length of the rectangle : ";
cin >> length ;
cout << "\n Enter the breadth of the rectangle : ";
cin >> breadth ;
}
float Enter_l(void)
{ return length ; }
}; // End of the class definition
class Rectangle1 : public Rectangle
{
private:
float area ;
public:
void Rec_area(void)
{ area = Enter_l( ) * breadth ; }
// area = length * breadth ; can't be used here
void Display(void)
{
cout << "\n Length = " << Enter_l( ) ; // Object of the derived class can't
// inherit the private member of the base class. Thus the member
// function is used here to get the value of data member 'length'.
cout << "\n Breadth = " << breadth ;
cout << "\n Area = " << area ;
}
}; // End of the derived class definition D
void main(void)
{
Rectangle1 r1 ;
r1.Enter_lb( );
r1.Rec_area( );
r1.Display( );
}
Figure- Public Inheritance
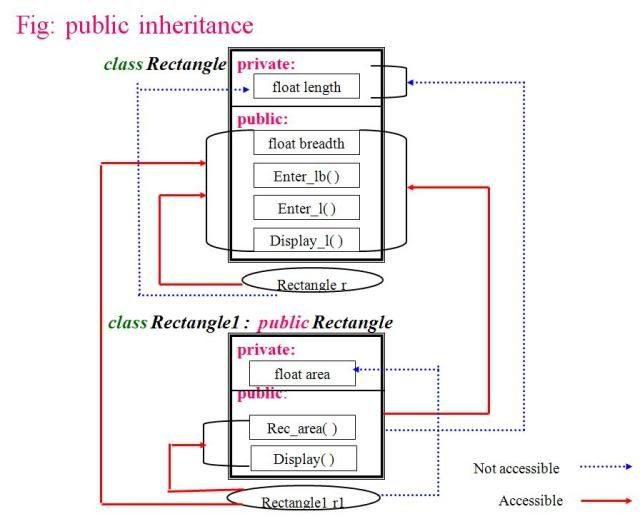
Private inheritance
When a derived class privately inherits a base class, all the public members of the base class become private for the derived class.
In this case, the public members of the base class can only be accessed by the member functions of the derived class.
The objects of the derived class cannot access the public members of the base class.
Note that whether the derived class is inherited publicly or privately from the base class, the private members of the base class cannot be inherited.
Example:
#include
#include
class Rectangle
{
int length, breadth;
public:
void enter()
{
cout << "\n Enter length: "; cin >> length;
cout << "\n Enter breadth: "; cin >> breadth;
}
int getLength()
{
return length;
}
int getBreadth()
{
return breadth;
}
void display()
{
cout << "\n Length= " << length;
cout << "\n Breadth= " << breadth;
}
};
class RecArea : private Rectangle
{
public:
void area_rec()
{
enter();
cout << "\n Area = " << (getLength() * getBreadth());
}
};
void main()
{
clrscr();
RecArea r ;
r.area_rec();
getch();
}
Example:2
#include
class Rectangle // Base class
{
private:
float length ; // This data member can't be inherited
public:
float breadth ; // This data member is inheritable
void Enter_lb(void)
{
cout << "\n Enter the length of the rectangle: "; cin >> length ;
cout << "\n Enter the breadth of the rectangle: "; cin >> bredth ;
}
float Enter_l(void)
{
return length ;
}
void Display_l(void)
{
cout << "\n Length = " << length ;
}
}; // End of the base class Rectangle.
// Defining the derived class Rectangle1. This class has been derived from the
// base class i.e. Rectangle, privately.
class Rectangle1 : private Rectangle // All the public members of the base class
{ // Rectangle become private for the derived class Rectangle1.
private:
float area ;
public:
void Rec_area(void)
{
Enter_lb( );
area = Enter_l( ) * bredth ; // length can't be used directly
}
void Display(void)
{
Display_l( ); // Displays the value of length.
cout << "\n Bredth = " << bredth ;
cout << "\n Area = " << area << endl ;
}
};
void main(void)
{
Rectangle1 r1 ;
r1.Rec_area( ) ;
// r.Enter_lb( ); will not work as it has become private for the derived class.
r1.Display( ) ;
// r.Display_l( ) will not work as it also has become private for the derived class.
}
Fig: private inheritance
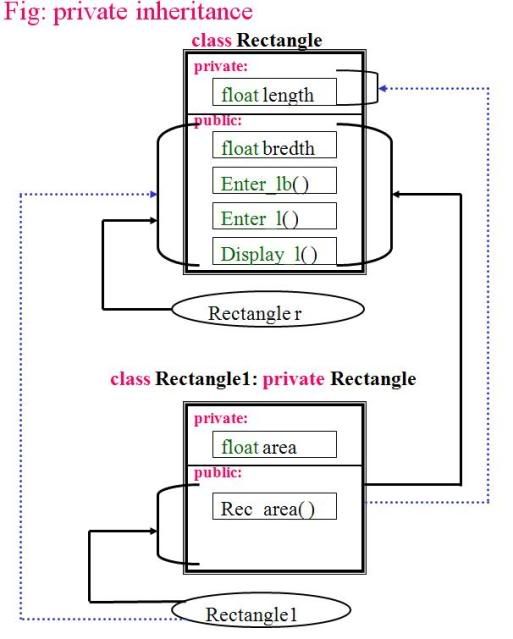
The protected access specifier
The third access specifier provided by C++ is protected.
The members declared as protected can be accessed by the member functions within their own class and any other class immediately derived from it.
These members cannot be accessed by the functions outside these two classes.
Therefore, the objects of the derived class cannot access protected members of the base class.
When the protected members (data, functions) are inherited in public mode, they become protected in the derived class. Thus, they can be accessed by the member functions of the derived class.
On other hand, if the protected members are inherited in the private mode, the members also become private in the derived class.
They can also be accessed by the member functions of the derived class, but cannot be inherited further.
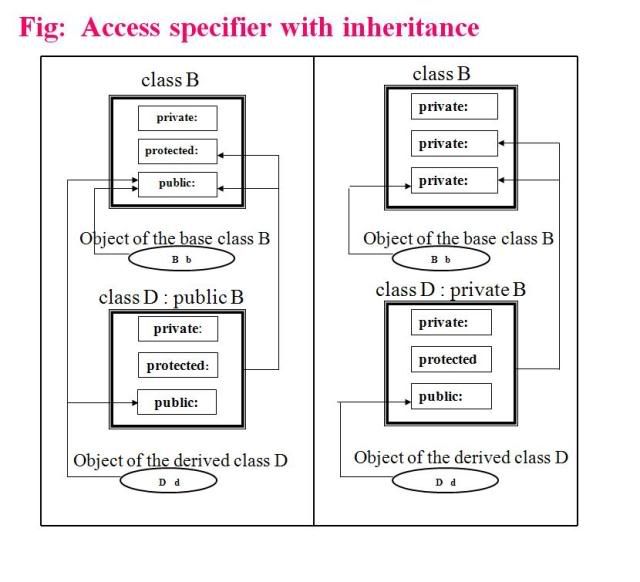
Overriding the member functions:
The member functions can also be used in a derived class, with the same name as those in the base class.
One might want to do this so that calls in the program work the same way for objects of both base and derived classes.
The following program will illustrate this concept.
#include
const int len = 20 ;
class Employee
{
private:
char F_name[len];
int I_number ;
int age ;
float salary ;
public:
void Enter_data(void)
{
cout << "\n Enter the first name = " ; cin >> F_name ;
cout << "\n Enter the identity number = " ; cin >> I_number ;
cout << "\n Enter the age = " ; cin >> age ;
cout << "\n Enter the salary = " ; cin >> salary ;
}
void Display_data(void)
{
cout << "\n Name = " << F_name ;
cout << "\n Identity Number = " << I_number ;
cout << "\n Age = " << age ;
cout << "\n Salary = " << salary ;
}
}; // End of the base class
class Engineer : public Employee
{
private:
char design[len] ; // S_Engineer, J_Engineer, Ex_Engineer etc
public:
void Enter_data( )
{
Employee :: Enter_data( ) ; // Overriding of the member function
cout << "\n Enter the designation of the Engineer: " ; cin >> design ;
}
void Display_data(void)
{
cout << "\n *******Displaying the particulars of the Engineer**** \n" ;
Employee :: Display_data( ) ; // Overriding of the member function
cout << "\n Designition = " << design ;
}
}; // End of the derived class
void main(void)
{
Engineer er ;
er.Enter_data( ) ;
er.Display_data( );
}
Hierarchical Inheritance:
When two or more classes are derived from a single base class, then
Inheritance is called the hierarchical inheritance. The representation
of the hierarchical inheritance is shown in the following Fig.
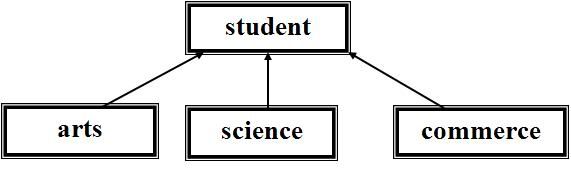
In the above Fig., student is a base class, from which the three classes viz. arts, science and commerce have been derived. Now, let us write a program that illustrates the hierarchical inheritance, based on the above design.
Example
include
const int len = 20 ;
class student // Base class
{
private: char F_name[len] , L_name[len] ;
int age, int roll_no ;
public:
void Enter_data(void)
{
cout << "\n\t Enter the first name: " ; cin >> F_name ;
cout << "\t Enter the second name: "; cin >> L_name ;
cout << "\t Enter the age: " ; cin >> age ;
cout << "\t Enter the roll_no: " ; cin >> roll_no ;
}
void Display_data(void)
{
cout << "\n\t First Name = " << F_name ;
cout << "\n\t Last Name = " << L_name ;
cout << "\n\t Age = " << age ;
cout << "\n\t Roll Number = " << roll_no ;
}
};
class arts : public student
{
private:
char asub1[len] ;
char asub2[len] ;
char asub3[len] ;
public:
void Enter_data(void)
{
student :: Enter_data( );
cout << "\t Enter the subject1 of the arts student: "; cin >> asub1 ;
cout << "\t Enter the subject2 of the arts student: "; cin >> asub2 ;
cout << "\t Enter the subject3 of the arts student: "; cin >> asub3 ;
}
void Display_data(void)
{
student :: Display_data( );
cout << "\n\t Subject1 of the arts student = " << asub1 ;
cout << "\n\t Subject2 of the arts student = " << asub2 ;
cout << "\n\t Subject3 of the arts student = " << asub3 ;
}
};
class commerce : public student
{
private: char csub1[len], csub2[len], csub3[len] ;
public:
void Enter_data(void)
{
student :: Enter_data( );
cout << "\t Enter the subject1 of the commerce student: ";
cin >> csub1;
cout << "\t Enter the subject2 of the commerce student: ";
cin >> csub2 ;
cout << "\t Enter the subject3 of the commerce student: ";
cin >> csub3 ;
}
void Display_data(void)
{
student :: Display_data( );
cout << "\n\t Subject1 of the commerce student = " << csub1 ;
cout << "\n\t Subject2 of the commerce student = " << csub2 ;
cout << "\n\t Subject3 of the commerce student = " << csub3 ;
}
};
void main(void)
{
arts a ;
cout << "\n Entering details of the arts student\n" ;
a.Enter_data( );
cout << "\n Displaying the details of the arts student\n" ;
a.Display_data( );
science s ;
cout << "\n\n Entering details of the science student\n" ;
s.Enter_data( );
cout << "\n Displaying the details of the science student\n" ;
s.Display_data( );
commerce c ;
cout << "\n\n Entering details of the commerce student\n" ;
c.Enter_data( );
cout << "\n Displaying the details of the commerce student\n";
c.Display_data( );
}
Multiple Inheritance
When a class is inherited from more than one base class, it is known as multiple inheritance.
The syntax for defining a subclass, which is inheriting more than one classes is:
class Subclass : access_specifier Baseclass1,
access_specifier Baseclass2, ………
…….. access_specifier Baseclass_n
{
members of the derived class ;
};
The following figure illustrates the use of multiple inheritance.
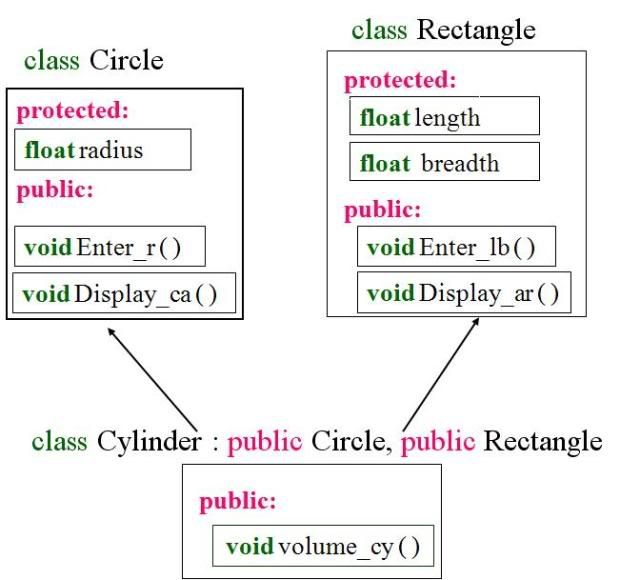
In the above figure, Circle and Rectangle are two base classes from which the class Cylinder is being inherited.
The data members of both the base classes are declared in protected mode. Thus, the class Cylinder can access the data member radius of class Circle and data member length, breadth of the class Rectangle, but the objects of the class Cylinder cannot access these protected data members.
The volume of the cylinder is equal to 22/7*(radius*radius*length). Thus, instead of defining these data again, they can be inherited from the base classes Circle and Rectangle ( radius from class Circle and length from class Rectangle ).
Example:
#include
class Circle // First base class
{
protected:
float radius ;
public:
void Enter_r(void)
{
cout << "\n\t Enter the radius: "; cin >> radius ;
}
void Display_ca(void)
{
cout << "\t The area = " << (22/7 * radius*radius) ;
}
};
class Rectangle // Second base class
{
protected:
float length, breadth ;
public:
void Enter_lb(void)
{
cout << "\t Enter the length : "; cin >> length ;
cout << “\t Enter the breadth : ” ; cin >> breadth ;
}
void Display_ar(void)
{
cout << "\t The area = " << (length * breadth);
}
};
class Cylinder : public Circle, public Rectangle // Derived class, inherited
{ // from classes Circle & Rectangle
public:
void volume_cy(void)
{
cout << "\t The volume of the cylinder is: "
<< (22/7* radius*radius*length) ;
}
};
void main(void)
{
Circle c ;
cout << "\n Getting the radius of the circle\n" ;
c.Enter_r( );
c.Display_ca( );
Rectangle r ;
cout << "\n\n Getting the length and breadth of the rectangle\n\n";
r.Enter_l( );
r.Enter_b( );
r.Display_ar( );
Cylinder cy ; // Object cy of the class cylinder which can access all the
// public members of the class circle as well as of the class rectangle
cout << "\n\n Getting the height and radius of the cylinder\n";
cy.Enter_r( );
cy.Enter_lb( );
cy.volume_cy( );
}
Multilevel Inheritance:
It has been discussed so far that a class can be derived from a class.
C++ also provides the facility of multilevel inheritance, according to which the derived class can also be derived by an another class, which in turn can further be inherited by another and so on.
The following figure will illustrate the meaning of the multilevel inheritance.
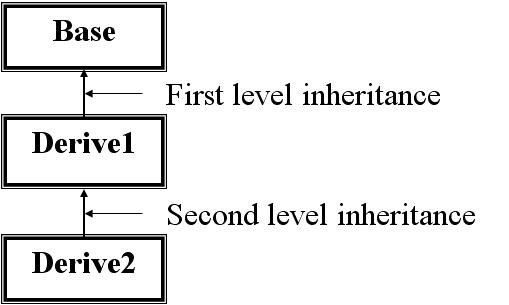
In the above figure, class B represents the base class. The class D1 that is called first level of inheritance, inherits the class B. The derived class D1 is further inherited by the class D2, which is called second level of inheritance.
Example: Multilevel Inheritance
#include
class Base
{
protected:
int b;
public:
void EnterData( )
{
cout << "\n Enter the value of b: ";
cin >> b;
}
void DisplayData( )
{
cout << "\n b = " << b;
}
};
class Derive1 : public Base
{
protected:
int d1;
public:
void EnterData( )
{
Base:: EnterData( );
cout << "\n Enter the value of d1: ";
cin >> d1;
}
void DisplayData( )
{
Base::DisplayData();
cout << "\n d1 = " << d1;
}
};
class Derive2 : public Derive1
{
private:
int d2;
public:
void EnterData( )
{
Derive1::EnterData( );
cout << "\n Enter the value of d2: ";
cin >> d2;
}
void DisplayData( )
{
Derive1::DisplayData( );
cout << "\n d2 = " << d2;
}
};
int main( )
{
Derive2 objd2;
objd2.EnterData( );
objd2.DisplayData( );
return 0;
}
No comments:
Post a Comment